So as we get some basic idea about structure now we define the structure and the structure name is Employee( you can change it) and its variable is ID, Name, Age, and Salary, as you can see that now our structure looks like the above syntax of the structure. Now the next step is to take the user input and store the user input value in structure with the help of 'For Loop' (you can use while and do while loops).
At the end we have to print the value stored in the structure to the console this will help to check whether your structure is performing well or not. Here I use iomanip for manipulating data better like while printing the data in a structured systematic manner and the setw() is used to print the data in a manner
Setw()
Sets the field width to be used on output operations, it comes under the iomainp header file.
Try This: C++ Program For Store Book Details Using Structure
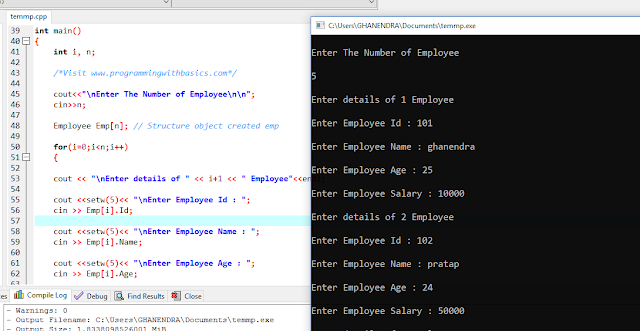
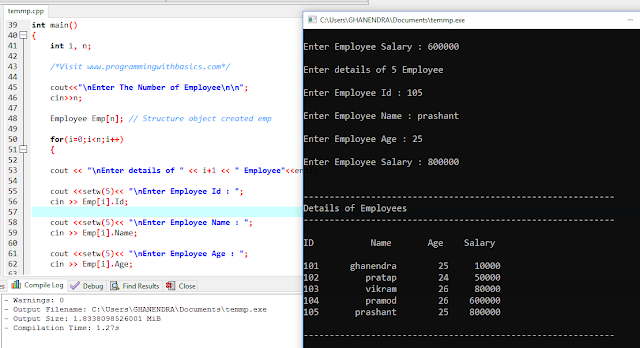
The structure is a user-defined data type in C++. The structure is used to represent a record. Suppose you want to store a record of a Student which consists of the student's name, address, roll number and age. You can define a structure to hold this information.
The struct keyword is used to define a structure. struct define a new data type which is a collection of different type of data.
Syntax:
struct structure_name
{
Statement...........1
Statement...........2
Statement...........3
.
.
.
Statement...........n
};
Note: here statement refers to variables and members of the structure.
Setw()
Sets the field width to be used on output operations, it comes under the iomainp header file.
Try This: C++ Program For Store Book Details Using Structure
C++ Program For Employee Details Using Structure
#include <iostream>
#include <iomanip>
using namespace std;
struct Employee
{
int Id;
char Name[25];
int Age;
long Salary;
};
int main()
{
int i, n;
/*Write a C++ Program to Get and Display Employee Details Using Structure
*/
cout << "\nEnter The Number of Employee\n\n";
cin >> n;
Employee Emp[n]; // Structure object created emp
for (i = 0; i < n; i++)
{
cout << "\nEnter Employee Details of " << i + 1 << " Employee" << endl;
cout << setw(5) << "\nEnter Employee ID: ";
cin >> Emp[i].Id;
cout << setw(5) << "\nEnter Employee Name: ";
cin >> Emp[i].Name;
cout << setw(5) << "\nEnter Employee Age: ";
cin >> Emp[i].Age;
cout << setw(5) << "\nEnter Employee Salary: ";
cin >> Emp[i].Salary;
}
cout << "\n\n------------------------------------------------------------\n";
cout << "Display Employee Details";
cout << "\n------------------------------------------------------------\n\n";
cout << "ID" << setw(15) << "Name" << setw(10) << "Age" << setw(10) << "Salary";
cout << endl;
for (i = 0; i < n; i++)
{
cout << "\n" << Emp[i].Id << setw(15) << Emp[i].Name << setw(10) << Emp[i].Age << setw(10) << Emp[i].Salary;
}
cout << "\n\n------------------------------------------------------------\n";
}
The Output in Employee Details Using Structure in C++
What Is Structure
The structure is a user-defined data type in C++. The structure is used to represent a record. Suppose you want to store a record of a Student which consists of the student's name, address, roll number and age. You can define a structure to hold this information.
Defining a Structure
The struct keyword is used to define a structure. struct define a new data type which is a collection of different type of data.
Syntax:
struct structure_name
{
Statement...........1
Statement...........2
Statement...........3
.
.
.
Statement...........n
};
Note: here statement refers to variables and members of the structure.
Similar to Employee Details Using Structure
- C++ Program To Print The Fibonacci Series Up to the Given Number Of Terms
- C++ Program to Sort Elements in Lexicographical Order (Dictionary Order) Using For Loop
- C++ Program To Find The GCD And LCM Of Two Numbers
- C++ Program To Find Whether A Number Is Palindrome Or Not
- C++ Program For Denomination of an Amount Using While Loop
0 Comments: