School management system project in java with source code free download pdf. This project has five sections first student management, lab management, staff management, accessories management and account management. There are subsections for each main section. Student management has 4 subsections add student details, display student details, delete student details and cancel or delete the student data from the student management system.
Similarly, lab management has item name, item serial number, cost of the item and item type. Staf section consists of the staff name, father name, mother name, qualification, date of birth, registration number and mobile number of the staff.
The accessories detail section has the accessories' names, accessories cost, purchase date and quantity. In our list, the last balance section has three subsections the first one is added balance, due balance and total balance. We have added one more section which is an about page of the college management system.
How To Run Java Applets Programs Using Command Line
- Create one separate folder at any location, I suggest creating a folder on the desktop.
- Open a notepad and copy the code and save the given code as sms.java
- Open cmd and go to your project location then run the program as javac sms.java and hit enter
- Again type appletviewer sms.java You Did It. he Code Will Work 100%
For more details follow this article: How to Run Java Applets and Swing Using Command Line


School Management System Project in Java With Source Code Free Download
import java.awt.*;
import java.awt.event.*;
import java.applet.*;
/*<applet code="sms" width=1500 height=1500></applet>*/
//*********APPLET CLASS***********
public class sms extends Applet implements ActionListener {
String msg = "*****WELCOME TO SCHOOL MANAGEMENT*****";
String msg2 = "";
String ss1, ss2, ss3, ss4, ss5, ss6, ss7;
String std1, std2, std3, std4, std5, std6, std7;
Image img;
int z = 0, z1 = 0, z2 = 0, z3 = 0, z4 = 0, z5 = 0, z6 = 0;
Button ab, bc, cd, de, ef, fg, gh;
//*********MAIN PAGE BUTTON**********
public void init() {
setLayout(null);
Font f = new Font("SansSerif", Font.BOLD, 22);
setFont(f);
ab = new Button("STUDENT MANAGEMENT");
ab.setBounds(50, 50, 350, 50);
add(ab);
bc = new Button("LAB MANAGEMENT");
bc.setBounds(100, 100, 350, 50);
add(bc);
cd = new Button("STAFF MANAGEMENT");
cd.setBounds(50, 150, 350, 50);
add(cd);
de = new Button("ACCESSORIES MANAGEMENT");
de.setBounds(100, 200, 350, 50);
add(de);
ef = new Button("ACCOUNT MANAGEMENT");
ef.setBounds(50, 250, 350, 50);
add(ef);
fg = new Button("ABOUT");
fg.setBounds(100, 300, 350, 50);
add(fg);
gh = new Button("EXIT");
gh.setBounds(50, 350, 350, 50);
add(gh);
img = getImage(getCodeBase(), "main.jpg");
ab.addActionListener(this);
bc.addActionListener(this);
cd.addActionListener(this);
de.addActionListener(this);
ef.addActionListener(this);
fg.addActionListener(this);
gh.addActionListener(this);
}
//*****ACTION PERFORMED FOR MAIN PAGE******
public void actionPerformed(ActionEvent ae) {
String str = ae.getActionCommand();
if (str == "STUDENT MANAGEMENT") {
Frame f;
f = new Student1("STUDENT MANAGEMENT");
f.setVisible(true);
f.setSize(1500, 1000);
} else if (str == "LAB MANAGEMENT") {
Frame f;
f = new Lab1("LAB MANAGEMENT");
f.setVisible(true);
f.setSize(1366, 768);
} else if (str == "STAFF MANAGEMENT") {
Frame f;
f = new Staff1("STAFF MANAGEMENT");
f.setVisible(true);
f.setSize(1500, 1000);
} else if (str == "ACCESSORIES MANAGEMENT") {
Frame f;
f = new Accessories1("ACCESSORIES MANAGEMENT");
f.setVisible(true);
f.setSize(1500, 1000);
} else if (str == "ACCOUNT MANAGEMENT") {
Frame f;
f = new Account1("ACCOUNT MANAGEMENT");
f.setVisible(true);
f.setSize(1500, 1000);
} else if (str == "ABOUT") {
Frame f;
f = new About1("ABOUT");
f.setVisible(true);
f.setSize(1500, 1000);
} else if (str == "EXIT") {
Frame f;
f = new Exit1("EXIT");
f.setVisible(true);
f.setSize(1500, 1000);
}
}
public void paint(Graphics g) {
g.drawImage(img, 0, 0, 1366, 768, this);
g.drawString(msg, 500, 50);
g.drawRect(425, 18, 675, 50);
g.drawRect(426, 19, 676, 50);
}
//******FIRST FRAME AND OTHER BUTTON*******
class Student1 extends Frame implements ActionListener {
Button a1, b1, c1, d1;
Image img1;
Student1(String title) {
super(title);
//char ch = '*';
MyWindowAdapter adapter = new MyWindowAdapter(this);
addWindowListener(adapter);
setBackground(Color.CYAN);
setForeground(Color.RED);
setLayout(null);
Font f = new Font("SansSerif", Font.BOLD, 30);
setFont(f);
a1 = new Button("ADD STUDENT");
b1 = new Button("DISPLAY");
c1 = new Button("DELETE");
d1 = new Button("CANCEL");
a1.setBackground(Color.yellow);
b1.setBackground(Color.yellow);
c1.setBackground(Color.yellow);
d1.setBackground(Color.yellow);
a1.setBounds(50, 100, 300, 50);
b1.setBounds(100, 150, 300, 50);
c1.setBounds(50, 200, 300, 50);
d1.setBounds(100, 250, 300, 50);
add(a1);
add(b1);
add(c1);
add(d1);
a1.addActionListener(this);
b1.addActionListener(this);
c1.addActionListener(this);
d1.addActionListener(this);
}
//******INSIDE FIRST FRAME NEXT FRAME BUTTON CODING******
public void actionPerformed(ActionEvent ae) {
String str = ae.getActionCommand();
if (str == "ADD STUDENT") { //this.setVisible(false);
Frame f;
f = new Student2("******** ENTER YOUR INFORMATION *******");
f.setVisible(true);
f.setSize(1500, 1000);
} else if (str == "DISPLAY") {
Frame f;
f = new StudentDisplay("******** STUDENT INFORMATION DISPLAY *******");
f.setVisible(true);
f.setSize(1500, 1000);
} else if (str == "DELETE") {
Frame f;
f = new Student1("******** STUDENT INFORMATION DELETE *******");
f.setVisible(false);
f.setSize(1500, 1000);
} else if (str == "CANCEL") {
Frame f;
f = new Student1("******** ENTER YOUR INFORMATION *******");
f.setVisible(false);
f.setSize(1500, 1000);
}
}
public void paint(Graphics g) {
g.drawImage(img1, 0, 0, 1366, 768, this);
}
}
//*****SECOND FRAME AND OTHER BUTTON*****
class Lab1 extends Frame implements ActionListener {
Button a1, b1, c1, d1;
Image img1;
Lab1(String title) {
super(title);
MyWindowAdapter1 adapter = new MyWindowAdapter1(this);
addWindowListener(adapter);
setBackground(Color.blue);
setForeground(Color.RED);
setLayout(null);
Font f = new Font("SansSerif", Font.BOLD, 30);
setFont(f);
a1 = new Button("ADD ITEM");
b1 = new Button("DISPLAY ITEM");
c1 = new Button("REMOVE ITEM");
d1 = new Button("CANCEL");
a1.setBackground(Color.yellow);
b1.setBackground(Color.yellow);
c1.setBackground(Color.yellow);
d1.setBackground(Color.yellow);
a1.setBounds(50, 100, 300, 50);
b1.setBounds(100, 150, 300, 50);
c1.setBounds(50, 200, 300, 50);
d1.setBounds(100, 250, 300, 50);
add(a1);
add(b1);
add(c1);
add(d1);
a1.addActionListener(this);
b1.addActionListener(this);
c1.addActionListener(this);
d1.addActionListener(this);
}
//****INSIDE SECOND FRAME NEXT FRAME BUTTON CODING****
public void actionPerformed(ActionEvent ae) {
String str = ae.getActionCommand();
if (str == "ADD ITEM") {
this.setVisible(false);
Frame f;
f = new Lab2("******** ENTER YOUR INFORMATION *******");
f.setVisible(true);
f.setSize(1500, 1000);
} else if (str == "DISPLAY ITEM") {
Frame f;
f = new Student1("******** LAB INFORMATION DISPLAY *******");
f.setVisible(false);
f.setSize(1500, 1000);
} else if (str == "REMOVE ITEM") {
Frame f;
f = new Student1("******** STUDENT INFORMATION DELETE *******");
f.setVisible(false);
f.setSize(1500, 1000);
} else if (str == "CANCEL") {
Frame f;
f = new Student1("******** ENTER YOUR INFORMATION *******");
f.setVisible(false);
f.setSize(1500, 1000);
}
}
public void paint(Graphics g) {
//g.drawImage(img1,0,0,1366,768,this);
}
}
//******THIRD FRAME AND OTHER BUTTON*******
class Staff1 extends Frame implements ActionListener {
Button a1, b1, c1, d1;
Image img1;
Staff1(String title) {
super(title);
MyWindowAdapter2 adapter = new MyWindowAdapter2(this);
addWindowListener(adapter);
setBackground(Color.RED);
setForeground(Color.BLACK);
setLayout(null);
Font f = new Font("SansSerif", Font.BOLD, 30);
setFont(f);
a1 = new Button("NEW ENTRY");
b1 = new Button("DISPLAY");
c1 = new Button("DELETE");
d1 = new Button("CANCEL");
a1.setBackground(Color.yellow);
b1.setBackground(Color.yellow);
c1.setBackground(Color.yellow);
d1.setBackground(Color.yellow);
a1.setBounds(50, 100, 300, 50);
b1.setBounds(100, 150, 300, 50);
c1.setBounds(50, 200, 300, 50);
d1.setBounds(100, 250, 300, 50);
add(a1);
add(b1);
add(c1);
add(d1);
a1.addActionListener(this);
b1.addActionListener(this);
c1.addActionListener(this);
d1.addActionListener(this);
}
//*****INSIDE THIRD FRAME NEXT FRAME BUTTON CODING*****
public void actionPerformed(ActionEvent ae) {
String str = ae.getActionCommand();
if (str == "NEW ENTRY") { //this.setVisible(false);
Frame f;
f = new Staff2("******** ENTER YOUR INFORMATION *******");
f.setVisible(true);
f.setSize(1500, 1000);
} else if (str == "DISPLAY") {
Frame f;
f = new StaffDisplay("******** STAFF INFORMATION DISPLAY *******");
f.setVisible(true);
f.setSize(1500, 1000);
} else if (str == "DELETE") {
Frame f;
f = new Student1("******** STAFF INFORMATION DELETE *******");
f.setVisible(false);
f.setSize(1500, 1000);
} else if (str == "CANCEL") {
Frame f;
f = new Student1("******** ENTER YOUR INFORMATION *******");
f.setVisible(false);
f.setSize(1500, 1000);
}
}
public void paint(Graphics g) {
//g.drawImage(img1,0,0,1366,768,this);
}
}
//****FORTH FRAME AND OTHER BUTTON****
class Accessories1 extends Frame implements ActionListener {
Button a1, b1, c1, d1;
Image img1;
Accessories1(String title) {
super(title);
//char ch = '*';
MyWindowAdapter3 adapter = new MyWindowAdapter3(this);
addWindowListener(adapter);
setBackground(Color.magenta);
setForeground(Color.RED);
setLayout(null);
Font f = new Font("SansSerif", Font.BOLD, 30);
setFont(f);
a1 = new Button("ADD ACCESSORIES");
b1 = new Button("DISPLAY ACCESSORIES");
c1 = new Button("DELETE ACCESSORIES");
d1 = new Button("CANCEL");
a1.setBackground(Color.yellow);
b1.setBackground(Color.yellow);
c1.setBackground(Color.yellow);
d1.setBackground(Color.yellow);
a1.setBounds(50, 100, 400, 50);
b1.setBounds(100, 150, 400, 50);
c1.setBounds(50, 200, 400, 50);
d1.setBounds(100, 250, 400, 50);
add(a1);
add(b1);
add(c1);
add(d1);
a1.addActionListener(this);
b1.addActionListener(this);
c1.addActionListener(this);
d1.addActionListener(this);
}
//****INSIDE FORTH FRAME NEXT FRAME BUTTON CODING****
public void actionPerformed(ActionEvent ae) {
String str = ae.getActionCommand();
if (str == "ADD ACCESSORIES") {
this.setVisible(false);
Frame f;
f = new Accessories2("******** ENTER YOUR INFORMATION *******");
f.setVisible(true);
f.setSize(1500, 1000);
} else if (str == "DISPLAY ACCESSORIES") {
Frame f;
f = new Student1("******** ACCESSORIES INFORMATION DISPLAY *******");
f.setVisible(false);
f.setSize(1500, 1000);
} else if (str == "DELETE ACCESSORIES") {
Frame f;
f = new Student1("******** ACCESSORIES INFORMATION DELETE *******");
f.setVisible(false);
f.setSize(1500, 1000);
} else if (str == "CANCEL") {
Frame f;
f = new Student1("******** ENTER YOUR INFORMATION *******");
f.setVisible(false);
f.setSize(1500, 1000);
}
}
public void paint(Graphics g) {
//g.drawImage(img1,0,0,1366,768,this);
}
}
//*****FIFTH FRAME AND OTHER BUTTON*****
class Account1 extends Frame implements ActionListener {
Button a1, b1, c1, d1;
Image img1;
Account1(String title) {
super(title);
MyWindowAdapter4 adapter = new MyWindowAdapter4(this);
addWindowListener(adapter);
setBackground(Color.GREEN);
setForeground(Color.BLACK);
setLayout(null);
Font f = new Font("SansSerif", Font.BOLD, 30);
setFont(f);
a1 = new Button("ADD BALANCE");
b1 = new Button("CHECK BALANCE");
c1 = new Button("DEBIT BALANCE");
d1 = new Button("CANCEL");
a1.setBackground(Color.yellow);
b1.setBackground(Color.yellow);
c1.setBackground(Color.yellow);
d1.setBackground(Color.yellow);
a1.setBounds(50, 100, 400, 50);
b1.setBounds(100, 150, 400, 50);
c1.setBounds(50, 200, 400, 50);
d1.setBounds(100, 250, 400, 50);
add(a1);
add(b1);
add(c1);
add(d1);
a1.addActionListener(this);
b1.addActionListener(this);
c1.addActionListener(this);
d1.addActionListener(this);
}
//****INSIDE FIFTH FRAME NEXT FRAME BUTTON CODING****
public void actionPerformed(ActionEvent ae) {
String str = ae.getActionCommand();
if (str == "ADD BALANCE") {
this.setVisible(false);
Frame f;
f = new Account2("******** ENTER YOUR INFORMATION *******");
f.setVisible(true);
f.setSize(1500, 1000);
} else if (str == "CHECK BALANCE") {
Frame f;
f = new Student1("******** BALANCE INFORMATION DISPLAY *******");
f.setVisible(false);
f.setSize(1500, 1000);
} else if (str == "DEBIT BALANCE") {
Frame f;
f = new Student1("******** BALANCE INFORMATION DISPLAY *******");
f.setVisible(false);
f.setSize(1500, 1000);
} else if (str == "CANCEL") {
Frame f;
f = new Student1("******** ENTER YOUR INFORMATION *******");
f.setVisible(false);
f.setSize(1500, 1000);
}
}
public void paint(Graphics g) {
//g.drawImage(img1,0,0,1366,768,this);
}
}
//****SIXTH FRAME AND OTHER BUTTON****
class About1 extends Frame implements ActionListener {
Button a1, b1;
Image img1;
About1(String title) {
super(title);
//char ch = '*';
MyWindowAdapter5 adapter = new MyWindowAdapter5(this);
addWindowListener(adapter);
setForeground(Color.blue);
Font f = new Font("SansSerif", Font.BOLD, 23);
setFont(f);
setLayout(new BorderLayout());
add(new Button("ABOUT NITW"), BorderLayout.NORTH);
add(new Label(""), BorderLayout.SOUTH);
add(new Button("NEXT"), BorderLayout.EAST);
add(new Button("BACK"), BorderLayout.WEST);
String msg = "National Institute of Technology, Warangal (Deemed University) , " +
"\nformerly known as Regional Engineering College, was established in 1959." +
"\nPandit Jawaharlal Nehru laid the foundation stone for this institute on October 10, 1959," +
"\n the first in the chain of 30 NITs (formerly known as RECs) in the country." +
"\nThe Institute is well known for its dedicated faculty," +
"\nstaff and the state-of-the art infrastructure conducive to a healthy academic environment." +
"\nThe Institute is constantly striving to achieve higher levels of technical excellence. " +
"\n Evolving a socially relevant and yet internationally acceptable curriculum, " +
"\nimplementing innovative and effective teaching methodologies and focusing on " +
"\nthe wholesome development of the students are our concerns. " +
"\nThanks to UNESCO and UK assistance in the past, many developmental activities were undertaken." +
"\nThe World Bank Assistance under Technical Education Quality Improvement Programme " +
"\n(TEQIP during 2004-09) had been a timely help in the overall development of the Institute." +
"\nThe Institute currently has thirteen academic departments and a few advanced research " +
"\ndisciplines of engineering, pure sciences and management, " +
"\nwith nearly 100 laboratories organized in a unique pattern of functioning," +
"\n Auditorium, Student Activity Centre, Mega Computer Centre, Indoor Games Complex, big stadium, " +
"\nSeminar Halls with required infrastructure, Dispensary with state of art of facilities, etc. \n\n" +
" - GHANENDRA YADAV\n\n";
add(new TextArea(msg), BorderLayout.CENTER);
//Font f=new Font("SansSerif",Font.BOLD,30);
//setFont(f);
//a1= new Button("BACK");
//b1= new Button("NEXT");
//a1.setBackground(Color.yellow);
//b1.setBackground(Color.yellow);
//a1.setBounds(200,600,300,50);
//b1.setBounds(1000,600,300,50);
//add(a1);
//add(b1);
//a1.addActionListener(this);
//b1.addActionListener(this);
}
//*****INSIDE SIXTH FRAME NEXT FRAME BUTTON CODING****
public void actionPerformed(ActionEvent ae) {
String str = ae.getActionCommand();
if (str == "BACK") {
this.setVisible(false);
Frame f;
f = new Student1("******** ENTER YOUR INFORMATION *******");
f.setVisible(false);
f.setSize(1500, 1000);
} else if (str == "NEXT") {
Frame f;
f = new Student1("******** ENTER YOUR INFORMATION *******");
f.setVisible(false);
f.setSize(1500, 1000);
}
}
public void paint(Graphics g) {
//g.drawImage(img1,0,0,1366,768,this);
}
}
//****SEVENTH FRAME AND OTHER BUTTON****
class Exit1 extends Frame implements ActionListener {
Button a1;
Image img1;
Exit1(String title) {
super(title);
//char ch = '*';
MyWindowAdapter6 adapter = new MyWindowAdapter6(this);
addWindowListener(adapter);
setBackground(Color.CYAN);
setForeground(Color.blue);
setLayout(null);
Font f = new Font("SansSerif", Font.BOLD, 40);
setFont(f);
a1 = new Button("LAST CLICK PLEASE......");
a1.setBackground(Color.PINK);
a1.setBounds(400, 300, 500, 150);
add(a1);
a1.addActionListener(this);
}
//****INSIDE SEVENTH FRAME NEXT FRAME BUTTON CODING***
public void actionPerformed(ActionEvent ae) {
String str = ae.getActionCommand();
if (str == "LAST CLICK PLEASE......") {
this.setVisible(false);
Frame f;
f = new Exit2("******** HAVE A GREAT DAY *******");
f.setVisible(true);
f.setSize(1500, 1000);
}
}
public void paint(Graphics g) {
//g.drawImage(img1,0,0,1366,768,this);
}
}
//****THIRD FRAME FOR STUDENT DATA****
class Student2 extends Frame implements ActionListener {
TextField t1, t2, t3, t4, t5, t6, t7;
Button bb1;
Label l1, l2, l3, l4, l5, l6, l7;
String str1;
Student2(String title) {
super(title);
MyWindowAdapter7 adapter = new MyWindowAdapter7(this);
addWindowListener(adapter);
setLayout(null);
Font f = new Font("SansSerif", Font.BOLD, 20);
setFont(f);
setBackground(Color.red);
t1 = new TextField(20);
t1.setBounds(400, 50, 400, 50);
add(t1);
l1 = new Label("STUDENT NAME");
add(l1);
l1.setBounds(200, 50, 200, 50);
t2 = new TextField(20);
t2.setBounds(400, 120, 400, 50);
add(t2);
l2 = new Label("COURSE");
add(l2);
l2.setBounds(200, 120, 200, 50);
t3 = new TextField(20);
t3.setBounds(400, 190, 400, 50);
add(t3);
l3 = new Label("ROLL NO");
add(l3);
l3.setBounds(200, 190, 200, 50);
t4 = new TextField(20);
t4.setBounds(400, 260, 400, 50);
add(t4);
l4 = new Label("DEPT");
add(l4);
l4.setBounds(200, 260, 200, 50);
t5 = new TextField(20);
t5.setBounds(400, 330, 400, 50);
add(t5);
l5 = new Label("DOB");
add(l5);
l5.setBounds(200, 330, 200, 50);
t6 = new TextField(20);
t6.setBounds(400, 390, 400, 50);
add(t6);
l6 = new Label("REG.NO");
add(l6);
l6.setBounds(200, 390, 200, 50);
t7 = new TextField(20);
t7.setBounds(400, 460, 400, 50);
add(t7);
l7 = new Label("MOB NO");
add(l7);
l7.setBounds(200, 460, 200, 50);
bb1 = new Button("SUBMIT");
bb1.setBackground(Color.yellow);
bb1.setBounds(500, 530, 200, 50);
add(bb1);
bb1.addActionListener(this);
}
public void actionPerformed(ActionEvent ae) {
/*String str=ae.getActionCommand();
str1=t1.getText();
t4.setText(str1);
int aa1 =Integer.parseInt(str1);
str1=t2.getText();
int bb1=Integer.parseInt(str1);
int cc1=aa1+bb1;
str1=Integer.toString(cc1);
t3.setText(str1);*/
ss1 = t1.getText();
ss2 = t2.getText();
ss3 = t3.getText();
ss4 = t4.getText();
ss5 = t5.getText();
ss6 = t6.getText();
ss7 = t7.getText();
}
}
//*****THIRD FRAME FOR TEACHER DATA*****
class Staff2 extends Frame implements ActionListener {
TextField t1, t2, t3, t4, t5, t6, t7;
Button bb1;
Label l1, l2, l3, l4, l5, l6, l7;
Staff2(String title) {
super(title);
MyWindowAdapter8 adapter = new MyWindowAdapter8(this);
addWindowListener(adapter);
setLayout(null);
Font f = new Font("SansSerif", Font.BOLD, 20);
setFont(f);
setBackground(Color.pink);
t1 = new TextField(20);
t1.setBounds(400, 50, 400, 50);
add(t1);
l1 = new Label("TEACHER NAME");
add(l1);
l1.setBounds(200, 50, 200, 50);
t2 = new TextField(20);
t2.setBounds(400, 120, 400, 50);
add(t2);
l2 = new Label("FATHER NAME");
add(l2);
l2.setBounds(200, 120, 200, 50);
t3 = new TextField(20);
t3.setBounds(400, 190, 400, 50);
add(t3);
l3 = new Label("MOTHER NAME");
add(l3);
l3.setBounds(200, 190, 200, 50);
t4 = new TextField(20);
t4.setBounds(400, 260, 400, 50);
add(t4);
l4 = new Label("QUALIFICATION");
add(l4);
l4.setBounds(200, 260, 200, 50);
t5 = new TextField(20);
t5.setBounds(400, 330, 400, 50);
add(t5);
l5 = new Label("DOB");
add(l5);
l5.setBounds(200, 330, 200, 50);
t6 = new TextField(20);
t6.setBounds(400, 390, 400, 50);
add(t6);
l6 = new Label("REG.NO");
add(l6);
l6.setBounds(200, 390, 200, 50);
t7 = new TextField(20);
t7.setBounds(400, 460, 400, 50);
add(t7);
l7 = new Label("MOB NO");
add(l7);
l7.setBounds(200, 460, 200, 50);
bb1 = new Button("SUBMIT");
bb1.setBackground(Color.yellow);
bb1.setBounds(500, 530, 200, 50);
add(bb1);
bb1.addActionListener(this);
}
public void actionPerformed(ActionEvent ae) {
String str = ae.getActionCommand();
std1 = t1.getText();
std2 = t2.getText();
std3 = t3.getText();
std4 = t4.getText();
std5 = t5.getText();
std6 = t6.getText();
std7 = t7.getText();
}
}
//*****THIRD FRAME FOR LAB DATA*****
class Lab2 extends Frame implements ActionListener {
TextField t1, t2, t3, t4;
Button bb1;
Label l1, l2, l3, l4;
Lab2(String title) {
super(title);
MyWindowAdapter9 adapter = new MyWindowAdapter9(this);
addWindowListener(adapter);
setLayout(null);
Font f = new Font("SansSerif", Font.BOLD, 20);
setFont(f);
setBackground(Color.orange);
t1 = new TextField(20);
t1.setBounds(400, 50, 400, 50);
add(t1);
l1 = new Label("ITEM NAME");
add(l1);
l1.setBounds(200, 50, 200, 50);
t2 = new TextField(20);
t2.setBounds(400, 120, 400, 50);
add(t2);
l2 = new Label("SERIAL NO");
add(l2);
l2.setBounds(200, 120, 200, 50);
t3 = new TextField(20);
t3.setBounds(400, 190, 400, 50);
add(t3);
l3 = new Label("COST");
add(l3);
l3.setBounds(200, 190, 200, 50);
t4 = new TextField(20);
t4.setBounds(400, 260, 400, 50);
add(t4);
l4 = new Label("ITEM TYPE");
add(l4);
l4.setBounds(200, 260, 200, 50);
bb1 = new Button("SUBMIT");
bb1.setBackground(Color.yellow);
bb1.setBounds(500, 400, 200, 50);
add(bb1);
bb1.addActionListener(this);
}
public void actionPerformed(ActionEvent ae) {
String str = ae.getActionCommand();
}
}
//*****THIRD FRAME FOR ACCESSORIES DATA*****
class Accessories2 extends Frame implements ActionListener {
TextField t1, t2, t3, t4;
Button bb1;
Label l1, l2, l3, l4;
Accessories2(String title) {
super(title);
MyWindowAdapter10 adapter = new MyWindowAdapter10(this);
addWindowListener(adapter);
setLayout(null);
Font f = new Font("SansSerif", Font.BOLD, 18);
setFont(f);
setBackground(Color.blue);
t1 = new TextField(20);
t1.setBounds(400, 50, 400, 50);
add(t1);
l1 = new Label("ACCESSORIES NAME");
add(l1);
l1.setBounds(200, 50, 220, 50);
t2 = new TextField(20);
t2.setBounds(400, 120, 400, 50);
add(t2);
l2 = new Label("ACCESSORIES COST");
add(l2);
l2.setBounds(200, 120, 220, 50);
t3 = new TextField(20);
t3.setBounds(400, 190, 400, 50);
add(t3);
l3 = new Label("PURCHASE DATE");
add(l3);
l3.setBounds(200, 190, 220, 50);
t4 = new TextField(20);
t4.setBounds(400, 260, 400, 50);
add(t4);
l4 = new Label("QUANTITY");
add(l4);
l4.setBounds(200, 260, 220, 50);
bb1 = new Button("SUBMIT");
bb1.setBackground(Color.yellow);
bb1.setBounds(500, 400, 200, 50);
add(bb1);
bb1.addActionListener(this);
}
public void actionPerformed(ActionEvent ae) {
String str = ae.getActionCommand();
}
}
//****THIRD FRAME FOR ACCOUNT MANAGEMENT DATA****
class Account2 extends Frame implements ActionListener {
TextField t1, t2, t3;
Button bb1, bb2;
Label l1, l2, l3;
Account2(String title) {
super(title);
MyWindowAdapter11 adapter = new MyWindowAdapter11(this);
addWindowListener(adapter);
setLayout(null);
Font f = new Font("SansSerif", Font.BOLD, 20);
setFont(f);
setBackground(Color.YELLOW);
t1 = new TextField(20);
t1.setBounds(400, 50, 400, 50);
add(t1);
l1 = new Label("ADD BALANCE");
add(l1);
l1.setBounds(200, 50, 200, 50);
t2 = new TextField(20);
t2.setBounds(400, 120, 400, 50);
add(t2);
l2 = new Label("DUE BALANCE");
add(l2);
l2.setBounds(200, 120, 200, 50);
t3 = new TextField(20);
t3.setBounds(400, 190, 400, 50);
add(t3);
l3 = new Label("TOTAL BALANCE");
add(l3);
l3.setBounds(200, 190, 200, 50);
bb1 = new Button("ADD");
bb1.setBackground(Color.yellow);
bb1.setBounds(500, 300, 180, 50);
add(bb1);
bb1.addActionListener(this);
bb2 = new Button("SUBMIT");
bb2.setBackground(Color.yellow);
bb2.setBounds(700, 300, 200, 50);
add(bb2);
bb2.addActionListener(this);
}
public void actionPerformed(ActionEvent ae) {
String str = ae.getActionCommand();
}
}
//*****THIRD FRAME FOR EXI******
class Exit2 extends Frame implements ActionListener {
Button bb1;
Exit2(String title) {
super(title);
MyWindowAdapter12 adapter = new MyWindowAdapter12(this);
addWindowListener(adapter);
setLayout(null);
Font f = new Font("SansSerif", Font.BOLD, 50);
setFont(f);
setBackground(Color.green);
bb1 = new Button("GOOD BYE...!!!!");
bb1.setBackground(Color.WHITE);
bb1.setBounds(400, 300, 500, 200);
add(bb1);
}
public void actionPerformed(ActionEvent ae) {
String str = ae.getActionCommand();
}
}
//*****STUDENT INFORMATION DISPLAY****
class StudentDisplay extends Frame {
TextField t1, t2, t3, t4, t5, t6, t7;
Label l1, l2, l3, l4, l5, l6, l7;
StudentDisplay(String title) {
super(title);
MyWindowAdapter13 adapter = new MyWindowAdapter13(this);
addWindowListener(adapter);
setLayout(null);
Font f = new Font("SansSerif", Font.BOLD, 20);
setFont(f);
setBackground(Color.red);
t1 = new TextField(20);
t1.setBounds(400, 50, 400, 50);
add(t1);
l1 = new Label("STUDENT NAME");
add(l1);
l1.setBounds(200, 50, 200, 50);
t2 = new TextField(20);
t2.setBounds(400, 120, 400, 50);
add(t2);
l2 = new Label("COURSE");
add(l2);
l2.setBounds(200, 120, 200, 50);
t3 = new TextField(20);
t3.setBounds(400, 190, 400, 50);
add(t3);
l3 = new Label("ROLL NO");
add(l3);
l3.setBounds(200, 190, 200, 50);
t4 = new TextField(20);
t4.setBounds(400, 260, 400, 50);
add(t4);
l4 = new Label("DEPT");
add(l4);
l4.setBounds(200, 260, 200, 50);
t5 = new TextField(20);
t5.setBounds(400, 330, 400, 50);
add(t5);
l5 = new Label("DOB");
add(l5);
l5.setBounds(200, 330, 200, 50);
t6 = new TextField(20);
t6.setBounds(400, 390, 400, 50);
add(t6);
l6 = new Label("REG.NO");
add(l6);
l6.setBounds(200, 390, 200, 50);
t7 = new TextField(20);
t7.setBounds(400, 460, 400, 50);
add(t7);
l7 = new Label("MOB NO");
add(l7);
l7.setBounds(200, 460, 200, 50);
t1.setText(ss1);
t2.setText(ss2);
t3.setText(ss3);
t4.setText(ss4);
t5.setText(ss5);
t6.setText(ss6);
t7.setText(ss7);
}
}
//*****STAFF INFORMATION DISPLAY*******
class StaffDisplay extends Frame {
TextField t1, t2, t3, t4, t5, t6, t7;
Label l1, l2, l3, l4, l5, l6, l7;
StaffDisplay(String title) {
super(title);
MyWindowAdapter14 adapter = new MyWindowAdapter14(this);
addWindowListener(adapter);
setLayout(null);
Font f = new Font("SansSerif", Font.BOLD, 20);
setFont(f);
setBackground(Color.pink);
t1 = new TextField(20);
t1.setBounds(400, 50, 400, 50);
add(t1);
l1 = new Label("TEACHER NAME");
add(l1);
l1.setBounds(200, 50, 200, 50);
t2 = new TextField(20);
t2.setBounds(400, 120, 400, 50);
add(t2);
l2 = new Label("FATHER NAME");
add(l2);
l2.setBounds(200, 120, 200, 50);
t3 = new TextField(20);
t3.setBounds(400, 190, 400, 50);
add(t3);
l3 = new Label("MOTHER NAME");
add(l3);
l3.setBounds(200, 190, 200, 50);
t4 = new TextField(20);
t4.setBounds(400, 260, 400, 50);
add(t4);
l4 = new Label("QUALIFICATION");
add(l4);
l4.setBounds(200, 260, 200, 50);
t5 = new TextField(20);
t5.setBounds(400, 330, 400, 50);
add(t5);
l5 = new Label("DOB");
add(l5);
l5.setBounds(200, 330, 200, 50);
t6 = new TextField(20);
t6.setBounds(400, 390, 400, 50);
add(t6);
l6 = new Label("REG.NO");
add(l6);
l6.setBounds(200, 390, 200, 50);
t7 = new TextField(20);
t7.setBounds(400, 460, 400, 50);
add(t7);
l7 = new Label("MOB NO");
add(l7);
l7.setBounds(200, 460, 200, 50);
t1.setText(std1);
t2.setText(std2);
t3.setText(std3);
t4.setText(std4);
t5.setText(std5);
t6.setText(std6);
t7.setText(std7);
}
}
//****WINDOW ADAPTER FOR STUDENT1 CLASSS****
class MyWindowAdapter extends WindowAdapter {
Student1 s1;
public MyWindowAdapter(Student1 s1) {
this.s1 = s1;
}
public void windowClosing(WindowEvent we) {
s1.setVisible(false);
}
}
//*******WINDOW ADAPTER FOR LAB1 CLASSS**********
class MyWindowAdapter1 extends WindowAdapter {
Lab1 labo;
public MyWindowAdapter1(Lab1 labo) {
this.labo = labo;
}
public void windowClosing1(WindowEvent we) {
labo.setVisible(false);
}
}
//*****WINDOW ADAPTER FOR STAFF1 CLASSS******
class MyWindowAdapter2 extends WindowAdapter {
Staff1 stf2;
public MyWindowAdapter2(Staff1 stf2) {
this.stf2 = stf2;
}
public void windowClosing(WindowEvent we) {
stf2.setVisible(false);
}
}
//****WINDOW ADAPTER FOR ACCESSORIES1 CLASSS****
class MyWindowAdapter3 extends WindowAdapter {
Accessories1 acs1;
public MyWindowAdapter3(Accessories1 acs1) {
this.acs1 = acs1;
}
public void windowClosing(WindowEvent we) {
acs1.setVisible(false);
}
}
//****WINDOW ADAPTER FOR ACCOUNT1 CLASSS****
class MyWindowAdapter4 extends WindowAdapter {
Account1 acc1;
public MyWindowAdapter4(Account1 acc1) {
this.acc1 = acc1;
}
public void windowClosing(WindowEvent we) {
acc1.setVisible(false);
}
}
//****WINDOW ADAPTER FOR ABOUT1 CLASSS*****
class MyWindowAdapter5 extends WindowAdapter {
About1 abt1;
public MyWindowAdapter5(About1 abt1) {
this.abt1 = abt1;
}
public void windowClosing(WindowEvent we) {
abt1.setVisible(false);
}
}
//****WINDOW ADAPTER FOR EXIT1 CLASSS*******
class MyWindowAdapter6 extends WindowAdapter {
Exit1 ex1;
public MyWindowAdapter6(Exit1 ex1) {
this.ex1 = ex1;
}
public void windowClosing(WindowEvent we) {
ex1.setVisible(false);
}
}
//****WINDOW ADAPTER FOR STUDENT2 CLASSS******
class MyWindowAdapter7 extends WindowAdapter {
Student2 ss1;
public MyWindowAdapter7(Student2 ss1) {
this.ss1 = ss1;
}
public void windowClosing(WindowEvent we) {
ss1.setVisible(false);
}
}
//*****WINDOW ADAPTER FOR STAFF2 CLASSS********
class MyWindowAdapter8 extends WindowAdapter {
Staff2 stff1;
public MyWindowAdapter8(Staff2 stff1) {
this.stff1 = stff1;
}
public void windowClosing(WindowEvent we) {
stff1.setVisible(false);
}
}
//*****WINDOW ADAPTER FOR LAB2 CLASSS*******
class MyWindowAdapter9 extends WindowAdapter {
Lab2 lab1;
public MyWindowAdapter9(Lab2 lab1) {
this.lab1 = lab1;
}
public void windowClosing(WindowEvent we) {
lab1.setVisible(false);
}
}
//***WINDOW ADAPTER FOR ACCESSORIES2 CLASSS*****
class MyWindowAdapter10 extends WindowAdapter {
Accessories2 ace2;
public MyWindowAdapter10(Accessories2 ace2) {
this.ace2 = ace2;
}
public void windowClosing(WindowEvent we) {
ace2.setVisible(false);
}
}
//***WINDOW ADAPTER FOR ACCOUNT2 CLASSS****
class MyWindowAdapter11 extends WindowAdapter {
Account2 acc2;
public MyWindowAdapter11(Account2 acc2) {
this.acc2 = acc2;
}
public void windowClosing(WindowEvent we) {
acc2.setVisible(false);
}
}
//****WINDOW ADAPTER FOR EXIT2 CLASSS*****
class MyWindowAdapter12 extends WindowAdapter {
Exit2 ex2;
public MyWindowAdapter12(Exit2 ex2) {
this.ex2 = ex2;
}
public void windowClosing(WindowEvent we) {
ex2.setVisible(false);
}
}
//**WINDOW ADAPTER FOR STUDENT DISPLAY CLASSS**
class MyWindowAdapter13 extends WindowAdapter {
StudentDisplay sd;
public MyWindowAdapter13(StudentDisplay sd) {
this.sd = sd;
}
public void windowClosing(WindowEvent we) {
sd.setVisible(false);
}
}
//***WINDOW ADAPTER FOR STAFF DISPLAY CLASSS****
class MyWindowAdapter14 extends WindowAdapter {
StaffDisplay stfd;
public MyWindowAdapter14(StaffDisplay stfd) {
this.stfd = stfd;
}
public void windowClosing(WindowEvent we) {
stfd.setVisible(false);
}
}
//********* END OF PROGRAM ********
}
The output of the Student Management System Project
4. STUDENT DATA DISPLAY
5. LAB DETAIL
6. STAFF DETAIL
7. ACCESSORIES DETAIL
8. BALANCE DETAIL

9. ABOUT SMS
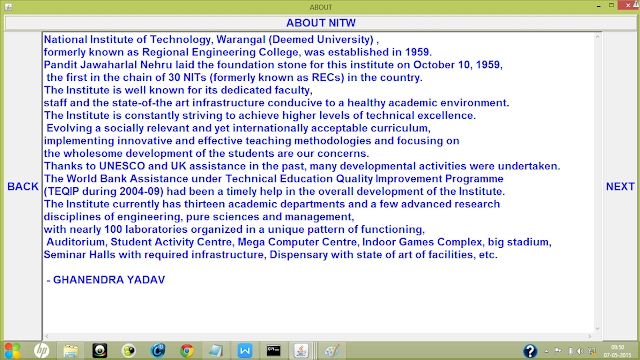
10. EXIT THE WINDOW

5. LAB DETAIL

6. STAFF DETAIL

7. ACCESSORIES DETAIL

8. BALANCE DETAIL

9. ABOUT SMS
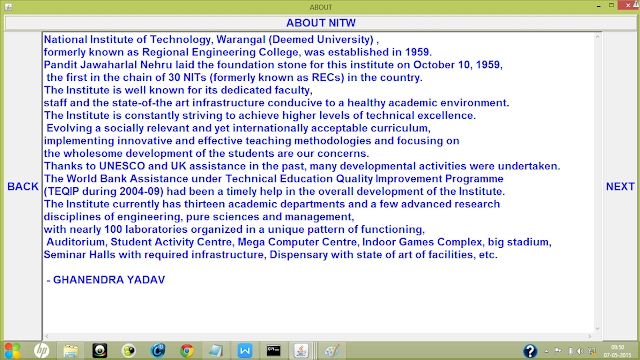
10. EXIT THE WINDOW

More Projects in C++
- C++ Program For Tic Tac Toe ( Game Project ) With Source Code
- C++ Program For School Management System ( SMS Project ) With Source Code
- C++ Program For HANGMAN ( GAME PROJECT ) With Source Code
- C++ Program For Casino Game: Number Guessing Program ( GAME PROJECT )
- C++ Program For Student Report Card ( SRC PROJECT ) With Source Code
More Projects in Java
- Student Management System Project in Java Using Multiple Classes
- Java Program For Calculator Using AWT Controls (GUI)
Resources
- Download A Code In PDF Click Here
- Download A Code In the JAVA File Click Here
- Download A Code With Proper SoftCopy Submission Click Here
0 Comments: