In this tutorial, we will understand Control flow in detail with complete syntax and examples but before starting tutorials I just want to clear up one doubt here in other programming languages we have only three JavaScript Control Flow (For Loop, Do…While Loop, and While Loop). We have one extra Control flow (For- in Loop), yes we have a total of four loops in javascript or we can say that four Control flow statements are in javascript.
Basically, control flow controls the flow of programming and with the help of Loop avoids repetitive code. So in these tutorials, we are going to learn the JavaScript Control Flow Structures or For Loop control flow javascript or For-In Loop control flow javascript or While Loop control flow javascript or Do-While control flow javascript in-depth with a complete explanation.
Types of Control Flow Statements in JavaScript
- Control Flow
- What is Loop
- Types of Loops
- For Loop
- For – in Loop
- While Loop
- Do-While Loop
- Syntax of Loops
- Examples of Loops
Note: I just want to clarify that there is a big difference between Control Flow and Conditional Statements tutorials in Javascript. If you don’t know about conditions statements I suggest you have to read the Conditional Statements in-depth article before reading this article. this will help you a lot to understand flow control flow in javascript.
1. Control Flow Statements
When a block of code needs to be executed many times with a different value, Then Control statements are required, According to a satisfied condition. Basically, Loops are there to avoid repetitive code.
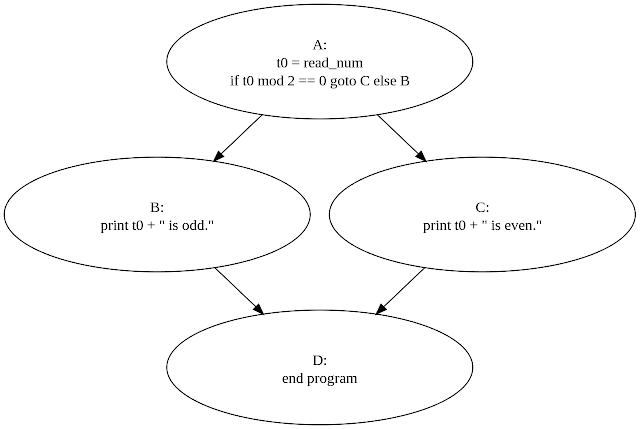
2. What are the Loops?
In computer programming, a loop is a sequence of instructions that is continually repeated until a certain condition is reached. Typically, a certain process is done, such as getting an item of data and changing it, and then some condition is checked such as whether a counter has reached a prescribed number or not.Types of Control Flow Statements in JavaScript
- For Loop
- For – in Loop
- While Loop
- Do-While Loop
1. For Loop Control Flow
For loop is there to execute a block of code multiple times, For Loop is one of the best examples of a control flow of programs in programming statements in Javascript. Below is the syntax of FOR LOOP
Syntax:
for (statement 1; statement 2; statement 3)
{
code block
}
Statement 1 is Checked or executed once before the execution.
Statement 2 Specify the condition of execution of code.
Statement 3 is executed every time until the specified condition is true.
Statement 2 Specify the condition of execution of code.
Statement 3 is executed every time until the specified condition is true.
Example:
for (i = 1; i <= 100; i++)
{
text += "Value of i is " + i + "<br>";
}
Here i=1 is statement1 which is executed once when the execution of for loop will be started. Basically, we will use this to initialize the variable used by the loop.
I <=100 is statement 2 which is the basic condition that statements will be executed until the value of I will is 100.
i++ is statement three which is executed whenever control satisfies the defined condition and I will increment by 1 every time.
I <=100 is statement 2 which is the basic condition that statements will be executed until the value of I will is 100.
i++ is statement three which is executed whenever control satisfies the defined condition and I will increment by 1 every time.
JavaScript Control Flow For Loop Example
<!DOCTYPE >
<html>
<body>
<h2>JavaScript Loops</h2>
<p id="ForLoopdemo"></p>
<script>
var number = "";
var n;
for (n = 0; n < 10; n++) {
number += " Number is " + n + "<br>";
}
document.getElementById("ForLoopdemo").innerHTML = number;
</script>
</body>
</html>
We can omit these statements:
1.) Statement 1 Can be Omitted:
You can omit statement 1 as it is optional for us, You can write it like this:
Example:
Initialize the variable before the loop started.
var Total = 0;
var i = 1;
for ( ; i < = 100; i++)
{
Total = Total + i;
}
alert("Sum = " + Total);
2.) Statement 2 Can be Omitted:
As statement 2 is optional loop will work fine but you can put a break inside the for loop otherwise it will execute infinite times. Initialize the variable before the loop started.
Example:
var Total = 0;
for (var i = 1 ; ; i++)
{
Total = Total + i;
Break;
}
alert("Sum = " + Total);
3.) Statement 3 Can be Omitted:
- If you don’t write statement 3 but it can be incremented inside the for a loop.
- if you don’t define any of the increments or decrements in the variable it will be executed only once.
- Initialize the variable before the loop started.
Example:
var Total = 0;
var i = 1;
for ( ; i < = 100;)
{
Total = Total + i;
i++;
}
alert("Sum = " + Total);
2. For-In Loop Control Flow
the for..in the loop is executed object’s properties.
Syntax:
for (variablename in object)
{
code Block;
}
here in For-In Loop each time one property of the object is assigned to the variable and the loop continues until the object’s properties are exhausted.
Example:
<!DOCTYPE html>
<html>
<body>
<p id="ForIn"></p>
<script>
var book = {AuthorName:"Suman", bookName:"Tutorial", age:25};
var bookProperties = "";
var attributes;
for (attributes in the book) {
bookProperties += book[attributes] + " ";
}
document.getElementById("ForIn").innerHTML = bookProperties;
</script>
</body>
</html>
3. Control Flow While Loop
while the loop will execute the block of code until the condition we specified is true. When the control comes to condition and found that the condition is false control comes out of the loop and executes the exact next statement after the while loop.
While Loop first checks the condition then statements are got executed.
while (var < lastvalue)
{
block of code;
}
Example:
<html>
<body>
<script type="text/javascript">
var n=0
while (n<10)
{
document.write("Value of n is " + n)
document.write("<br />")
n=n+1
}
</script>
</body>
</html>
Here loop will be executed 10 times, the value of the loop starts for n=0 and the condition will be true till n=9. When n=10 then the condition will be false and control comes out of the While loop.
4. Do…While Loop Control Flow Statements
The do…while loop, you can also say it is a variant of a while. Here the block of code will be executed at least once before testing the condition. So even if the condition becomes false though the code will be executed once.
do
{
Block of code;
}
while (var<=lastValue)
Example:
Tip: Always be careful when you use control flow in programming cause if your desired conditions are not matched then your control flow may be infinite or your loop can be endless. so always twice checks the conditions of Loops and you can fix the flow of a program using loops.
<html>
<body>
<script type="text/javascript">
var n=0
do
{
document.write("value of n is " + n)
document.write("<br />")
n=n+1
}
while (n<10)
</script>
</body>
</html>
Tip: Always be careful when you use control flow in programming cause if your desired conditions are not matched then your control flow may be infinite or your loop can be endless. so always twice checks the conditions of Loops and you can fix the flow of a program using loops.
Basic and well defined article.Good job.
ReplyDeleteThank You, You Like It. Please Keep visit and Share.
Delete