Problem :- Write A C++ Program To Find Greatest Among Three Numbers .Take three numbers from user and print the greatest number among them ex :- three numbers are 10 ,20 ,30 then 30 is greatest show 30 in output screen .
Logic :- For this problem there is a simple logic that take all three numbers and choose one number and compare that number to rest of the two number is number is greatest then print else choose second number and repeat the previous process if greatest then print second number is greatest else go to third number and repeat the same process .We are taking a Example and check all the process Step by Step
Example :- We are taking a Three number 55,65,75 as Input
Step 1 :- Select first number 55 and compare to 65 and 75 as we know 55 is not greater so control transfer to second condition .
Step 2 :- Now choose Second number 65 and compare to 55 and 75 as we know 65 is greater that 55 but not greater than 75 so control transfer to Third condition .
Step 3 :- Now choose Third number 75 and compare to 65 and 55 as we know 75 is greater compare both number 55 and 65 .Now we have a greatest number then print the number
Step 4 :- This is a Special Step if all condition didn't Match then All Number are equal .Example :- 50 ,50 ,50 then control goes to last condition and program Output is "All Are Equal "
Solution :-
Method 1:-
#include <stdio.h>
int main()
{
/* Program By Ghanendra Yadav
Visit http://www.programmingwithbasics.com/
*/
double num1, num2, num3;
printf("Enter Three Numbers: ");
scanf("%lf %lf %lf", &num1, &num2, &num3);
if (num1>=num2)
{
if(num1>=num3)
printf("%.2lf Is The Largest Number. ", num1);
else
printf("%.2lf Is The Largest Number. ", num3);
}
else
{
if(num2>=num3)
printf("%.2lf Is The Largest Number. ", num2);
else
printf("%.2lf is the largest number.",num3);
}
return 0;
}
Method 2:-
#include<stdio.h>
int main()
{
/* Program By Ghanendra Yadav
Visit http://www.programmingwithbasics.com/
*/
double num1, num2, num3;
printf("Enter Three Numbers: \n");
scanf("%lf %lf %lf", &num1, &num2, &num3);
if (num1 > num2 && num1 > num3)
printf("\nGreatest Number Is :%lf",num1);
else if (num2 > num3 && num2 > num1)
printf("\nGreatest Number Is :%lf",num2);
else if (num1 > num1 && num3 > num2)
printf("\nGreatest Number Is :%lf",num1);
else if ((num1==num2) && (num2==num3) && (num3==num1))
printf("\nAll Are Equal");
}
Output:-

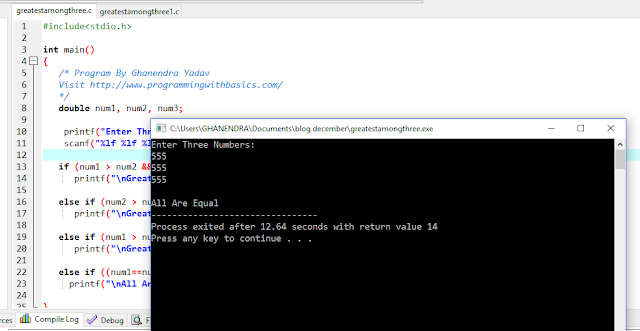
Logic :- For this problem there is a simple logic that take all three numbers and choose one number and compare that number to rest of the two number is number is greatest then print else choose second number and repeat the previous process if greatest then print second number is greatest else go to third number and repeat the same process .We are taking a Example and check all the process Step by Step
Example :- We are taking a Three number 55,65,75 as Input
Step 1 :- Select first number 55 and compare to 65 and 75 as we know 55 is not greater so control transfer to second condition .
Step 2 :- Now choose Second number 65 and compare to 55 and 75 as we know 65 is greater that 55 but not greater than 75 so control transfer to Third condition .
Step 3 :- Now choose Third number 75 and compare to 65 and 55 as we know 75 is greater compare both number 55 and 65 .Now we have a greatest number then print the number
Step 4 :- This is a Special Step if all condition didn't Match then All Number are equal .Example :- 50 ,50 ,50 then control goes to last condition and program Output is "All Are Equal "
See Also :- C++ Program To Find Greatest Among Three Numbers
Solution :-
Method 1:-
#include <stdio.h>
int main()
{
/* Program By Ghanendra Yadav
Visit http://www.programmingwithbasics.com/
*/
double num1, num2, num3;
printf("Enter Three Numbers: ");
scanf("%lf %lf %lf", &num1, &num2, &num3);
if (num1>=num2)
{
if(num1>=num3)
printf("%.2lf Is The Largest Number. ", num1);
else
printf("%.2lf Is The Largest Number. ", num3);
}
else
{
if(num2>=num3)
printf("%.2lf Is The Largest Number. ", num2);
else
printf("%.2lf is the largest number.",num3);
}
return 0;
}
Method 2:-
#include<stdio.h>
int main()
{
/* Program By Ghanendra Yadav
Visit http://www.programmingwithbasics.com/
*/
double num1, num2, num3;
printf("Enter Three Numbers: \n");
scanf("%lf %lf %lf", &num1, &num2, &num3);
if (num1 > num2 && num1 > num3)
printf("\nGreatest Number Is :%lf",num1);
else if (num2 > num3 && num2 > num1)
printf("\nGreatest Number Is :%lf",num2);
else if (num1 > num1 && num3 > num2)
printf("\nGreatest Number Is :%lf",num1);
else if ((num1==num2) && (num2==num3) && (num3==num1))
printf("\nAll Are Equal");
}
Output:-

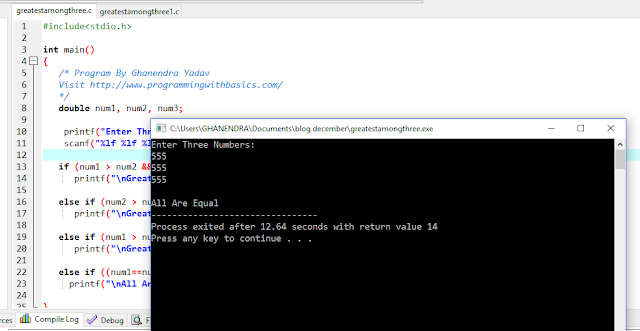
Now do it with 100 numbers.
ReplyDeleteUse Array For 100 Or n Numbers
Delete